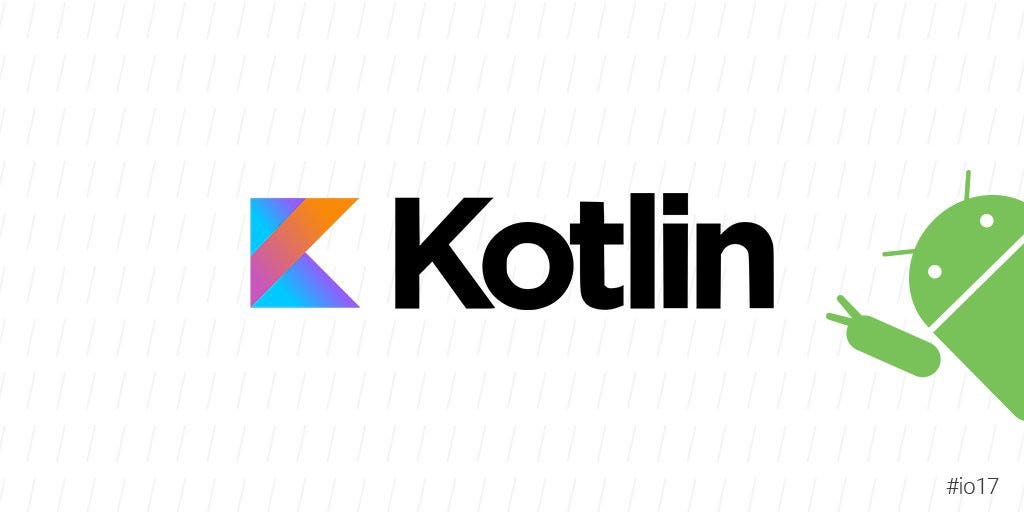
Master Kotlin Tricks: Expert Techniques for Efficient Coding
Introduction: Unleashing the Power of Kotlin Tricks
Alright, fellow coders, today we’re diving deep into the world of Kotlin tricks—those expert techniques that can take your coding skills from good to great. Kotlin is already a powerful language, but when you master these tricks, you’ll be able to write cleaner, more efficient, and more maintainable code. Let’s explore some of the top Kotlin tricks that every programmer should know.
Tip 1: Mastering the Art of Concise Syntax
One of the hallmarks of Kotlin is its concise syntax, and mastering this aspect can greatly improve your coding efficiency. With tricks like type inference, lambda expressions, and single-expression functions, you can reduce the amount of boilerplate code in your projects. This not only makes your code more readable but also saves you time and effort in the long run.
Tip 2: Leveraging the Power of Null Safety
Null pointer exceptions can be a nightmare for developers, but Kotlin’s null safety features make them a thing of the past. Tricks like safe calls (?.
), Elvis operator (?:
), and non-null assertions (!!
) allow you to handle null values with ease. By using these tricks, you can write code that is more robust and less prone to unexpected crashes.
Tip 3: Exploring the World of Extension Functions
Extension functions are a powerful Kotlin feature that allows you to add new functionality to existing classes without modifying their source code. With this trick, you can create utility functions that enhance the capabilities of standard library classes or even your own custom classes. This promotes code reuse and keeps your codebase clean and modular.
Tip 4: Unveiling the Secrets of Coroutines
Asynchronous programming is essential for building responsive and efficient applications, and Kotlin’s coroutines make it easier than ever. With tricks like async
, await
, and launch
, you can write asynchronous code in a sequential and straightforward manner. This improves the readability of your code and makes handling concurrency a breeze.
Tip 5: Harnessing the Power of Sealed Classes
Sealed classes are a versatile Kotlin trick that allows you to define restricted hierarchies of classes. They are particularly useful for implementing finite state machines, representing data structures with a fixed number of options, or handling different states in your application. By mastering sealed classes, you can write code that is more expressive and easier to understand.
Tip 6: Elevating Your Coding Game with Inline Functions
Inline functions are a Kotlin trick that allows you to improve the performance of your code by eliminating the overhead of function calls. When you mark a function as inline
, the compiler replaces the function call with the actual code at compile-time. This reduces the runtime overhead and can lead to faster and more efficient code execution.
Tip 7: Enhancing Code Readability with DSLs
Domain-specific languages (DSLs) are a powerful Kotlin trick that allows you to create custom syntax for specific tasks or domains. With DSLs, you can write code that is more expressive and closely resembles the problem domain you are working with. This improves the readability of your code and makes it easier for others to understand and maintain.
Tip 8: Boosting Efficiency with Lazy Initialization
Lazy initialization is a Kotlin trick that allows you to defer the creation of an object until it is actually needed. This can be particularly useful for expensive operations or resources that you don’t want to create until they are absolutely necessary. By using lazy initialization, you can improve the performance of your code and reduce memory usage.
Tip 9: Simplifying Code with Data Classes
Data classes are a Kotlin trick that allows you to create classes for storing data without writing boilerplate code. With data classes, you get automatic implementations of equals
, hashCode
, toString
, and copy
methods, making it easy to work with data objects. This trick reduces the amount of code you need to write and improves the maintainability of your projects.
Tip 10: Exploring Advanced Kotlin Libraries
Finally, to truly master Kotlin, it’s essential to explore and leverage the power of advanced Kotlin libraries and frameworks. Tricks like using Ktor for building web applications, Anko for Android development, or kotlinx.coroutines for asynchronous programming can greatly enhance your coding capabilities. By learning and using these libraries, you can build powerful and efficient applications with ease.
Conclusion: Becoming a Kotlin Trickster
There you have it, folks—ten top Kotlin tricks to take your coding skills to the next level. Whether you’re optimizing performance with inline functions, simplifying code with data classes, or exploring advanced Kotlin libraries, these tricks will help you write cleaner, more efficient, and more maintainable code. So go ahead, dive into Kotlin, master these tricks, and become a Kotlin trickster in no time! Read more about kotlin tricks